How to implement stack data structure from scratch?
Stack Data Structure Implementing Stack in C Push and Pop functions
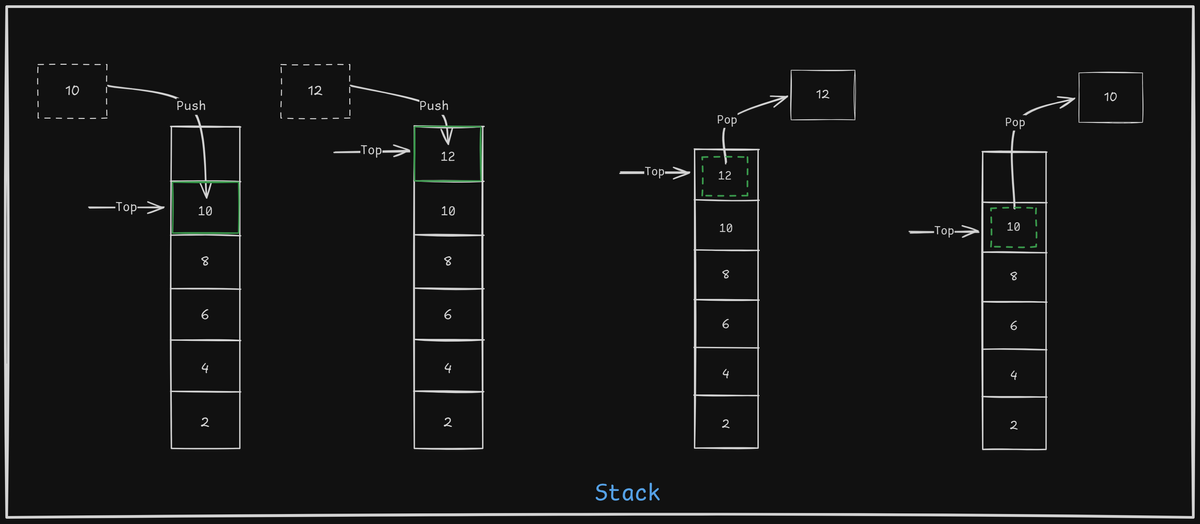
Stack data structure enables you to use LIFO [ Last IN first OUT ] approach. Whatever data comes first goes last. It also called FILO [ First IN Last OUT ]. For I/O, it provides you two functions.
- Push [ To insert data into stack ]
- Pop [ To delete the data from the stack ]
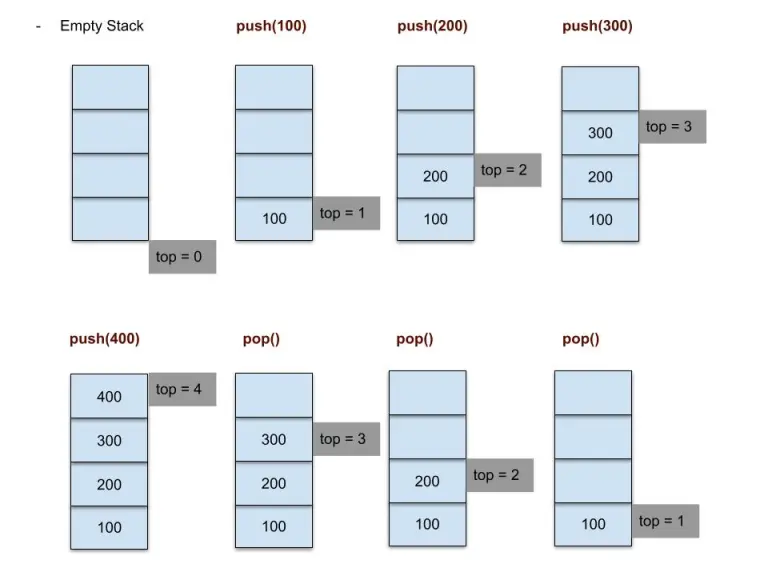
Have you ever thought about where this stack concept is being used?
All the functions call has LIFO approach.
How to create your own stack from scratch. The below code is just a sample of creating and utilizing a stack.
#ifndef __STACK_H__
#define __STACK_H__
typedef struct _stack
{
unsigned int *data;
unsigned int top;
unsigned int size;
}stack;
stack* createStack(unsigned int size_of_stack);
void push(stack *s, unsigned int val);
int pop(stack *s);
void destroyStack(stack *s);
void showStack(stack *s);
#endif
stack.h
#include <stdio.h>
#include <stdlib.h>
#include "stack.h"
stack* createStack(unsigned int size_of_stack)
{
stack *s = malloc(sizeof(stack));
s->data = malloc(sizeof(size_of_stack));
if(s->data == NULL){
free(s);
return NULL;
}
s->top = 0;
s->size = size_of_stack;
return s;
}
void push(stack *s, unsigned int val)
{
if(s->top == s->size){
printf("Stack is full\n");
return;
}
s->data[(s->top)++] = val;
}
int pop(stack *s)
{
if(s->top == 0){
printf("Stack is empty\n");
return -1;
}
return(s->data[--(s->top)]);
}
void destroyStack(stack *s)
{
if(s != NULL){
if(s->data != NULL){
free(s->data);
}
free(s);
}
}
void showStack(stack *s)
{
static int i =0;
if(i < s->top){
i = i+1;
showStack(s);
}
else{
return;
}
printf("[ %d ]\n",s->data[--i]);
}
stack.c
#include <stdio.h>
#include <stdlib.h>
#include "stack.h"
stack* createStack(unsigned int size_of_stack)
{
stack *s = malloc(sizeof(stack));
s->data = malloc(sizeof(size_of_stack));
if(s->data == NULL){
free(s);
return NULL;
}
s->top = 0;
s->size = size_of_stack;
return s;
}
void push(stack *s, unsigned int val)
{
if(s->top == s->size){
printf("Stack is full\n");
return;
}
s->data[(s->top)++] = val;
}
int pop(stack *s)
{
if(s->top == 0){
printf("Stack is empty\n");
return -1;
}
return(s->data[--(s->top)]);
}
void destroyStack(stack *s)
{
if(s != NULL){
if(s->data != NULL){
free(s->data);
}
free(s);
}
}
void showStack(stack *s)
{
static int i =0;
if(i < s->top){
i = i+1;
showStack(s);
}
else{
return;
}
printf("[ %d ]\n",s->data[--i]);
}
main.c